LineCollections¶
A LineCollection
is a container for emission lines which, much like an Sed
object does for spectra (see the `Sed
notebook <../sed/sed_example.ipynb>`__), provides a simple interface for complex operations on emission lines. In this notebook we detail how to work with LineCollection
objects.
Creating a LineCollection¶
Before demonstrating anything, we need a LineCollection
to demonstrate with. For this purpose, we will take one directly from a Grid
object (more information here). For more details on line generation see the grid lines and galaxy lines notebooks for thorough demonstrations of how to generate lines from each.
[1]:
from unyt import Myr
from synthesizer.grid import Grid
grid_dir = "../../../tests/test_grid"
grid_name = "test_grid"
grid = Grid(grid_name, grid_dir=grid_dir)
# Extract the lines for a specific point in the grid
lines = grid.get_lines(
grid.get_grid_point(log10ages=7.0 * Myr, metallicity=0.01)
)
Printing a summary of the LineCollection¶
If we want a summary of what a line contains we can simply print it. This will give us a table showing all the different attributes attached to a LineCollection
object and their values (or a summary of their values for large arrays).
[2]:
print(lines)
print(lines.line_ids)
+-------------------------------------------------------------------------------------------------+
| LINECOLLECTION |
+---------------------------+---------------------------------------------------------------------+
| Attribute | Value |
+---------------------------+---------------------------------------------------------------------+
| nlines | 215 |
+---------------------------+---------------------------------------------------------------------+
| ndim | 1 |
+---------------------------+---------------------------------------------------------------------+
| nlam | 215 |
+---------------------------+---------------------------------------------------------------------+
| shape | (215,) |
+---------------------------+---------------------------------------------------------------------+
| available_ratios | [BalmerDecrement, N2, S2, O1, R2, R3, R23, |
| | O32, Ne3O2] |
+---------------------------+---------------------------------------------------------------------+
| available_diagrams | [OHNO, BPT-NII, VO78-SII, VO78-OI] |
+---------------------------+---------------------------------------------------------------------+
| elements | [He, O, O, He, Si, Si, He, H, O, N, N, Si, |
| | Si, Si, O, O, O, C, C, C, Si, O, O, Si, O, |
| | O, N, Si, Si, C, C, Ne, He, O, C, O, O, Al, |
| | N, Mg, Si, Si, C, C, C, Si, O, C, C, Fe, Fe, |
| | Fe, Fe, C, Fe, Fe, Fe, Fe, Fe, Fe, Mg, Fe, |
| | Fe, Mg, Mg, Mg, Fe, Fe, Ar, Fe, He, Ne, Ne, |
| | Fe, Fe, O, O, H, H, Fe, H, H, H, Ne, He, H, |
| | Fe, Ne, H, S, S, H, Fe, Fe, Fe, Fe, H, Fe, |
| | Fe, Fe, Fe, O, Fe, Fe, Fe, Fe, Fe, He, Fe, |
| | H, Fe, Fe, Fe, Fe, Fe, O, Fe, Fe, Fe, O, Fe, |
| | Fe, Fe, Fe, Fe, Fe, Fe, Fe, Fe, Fe, Fe, Fe, |
| | Fe, Fe, Fe, Fe, Fe, Fe, Fe, Fe, He, He, Fe, |
| | O, O, Fe, N, H, N, Ni, He, S, S, Ar, Fe, Fe, |
| | Ca, Ca, Ni, Fe, Ni, Fe, Ar, O, O, O, Cl, Fe, |
| | Fe, Fe, S, Fe, H, Fe, Fe, Fe, S, H, H, S, |
| | S, S, He, He, H, O, O, O, O, O, Ni, Fe, Fe, |
| | H, Fe, Fe, Fe, Fe, Fe, Fe, Fe, Fe, Fe, Fe, |
| | Fe, Fe, Fe, Fe, H, Fe, Ni, Fe, Si, H, Si,] |
+---------------------------+---------------------------------------------------------------------+
| line_ids (215,) | [He 2 1025.27A, O 6 1031.91A, O 6 1037.61A, ...] |
+---------------------------+---------------------------------------------------------------------+
| lam (215,) | 1.03e+03 Å -> 2.48e+04 Å (Mean: 5.98e+03 Å) |
+---------------------------+---------------------------------------------------------------------+
| luminosity (215,) | 0.00e+00 erg/s -> 3.34e+34 erg/s (Mean: 3.52e+32 erg/s) |
+---------------------------+---------------------------------------------------------------------+
| continuum (215,) | 2.32e+17 erg/(Hz*s) -> 6.24e+19 erg/(Hz*s) (Mean: 1.62e+19 erg) |
+---------------------------+---------------------------------------------------------------------+
| cont (215,) | 2.32e+17 erg/(Hz*s) -> 6.24e+19 erg/(Hz*s) (Mean: 1.62e+19 erg) |
+---------------------------+---------------------------------------------------------------------+
| continuum_llam (215,) | 7.40e+28 erg/(s*Å) -> 2.52e+31 erg/(s*Å) (Mean: 5.76e+30 erg/(s*Å)) |
+---------------------------+---------------------------------------------------------------------+
| energy (215,) | 5.00e-01 eV -> 1.21e+01 eV (Mean: 3.76e+00 eV) |
+---------------------------+---------------------------------------------------------------------+
| equivalent_width (215,) | 0.00e+00 Å -> 7.09e+04 Å (Mean: 5.37e+02 Å) |
+---------------------------+---------------------------------------------------------------------+
| lum (215,) | 0.00e+00 erg/s -> 3.34e+34 erg/s (Mean: 3.52e+32 erg/s) |
+---------------------------+---------------------------------------------------------------------+
| nu (215,) | 1.21e+14 Hz -> 2.92e+15 Hz (Mean: 9.09e+14 Hz) |
+---------------------------+---------------------------------------------------------------------+
| vacuum_wavelengths (215,) | 1.03e+03 Å -> 2.48e+04 Å (Mean: 5.98e+03 Å) |
+---------------------------+---------------------------------------------------------------------+
['He 2 1025.27A' 'O 6 1031.91A' 'O 6 1037.61A' 'He 2 1084.94A'
'Si 2 1179.59A' 'Si 3 1206.50A' 'He 2 1215.13A' 'H 1 1215.67A'
'O 5 1218.34A' 'N 5 1238.82A' 'N 5 1242.80A' 'Si 2 1260.42A'
'Si 2 1264.74A' 'Si 2 1265.00A' 'O 1 1302.17A' 'O 1 1304.86A'
'O 1 1306.03A' 'C 2 1334.53A' 'C 2 1335.66A' 'C 2 1335.71A'
'Si 4 1393.75A' 'O 4 1399.78A' 'O 4 1401.16A' 'Si 4 1402.77A'
'O 4 1404.81A' 'O 4 1407.38A' 'N 4 1486.50A' 'Si 2 1526.71A'
'Si 2 1533.43A' 'C 4 1548.19A' 'C 4 1550.77A' 'Ne 4 1601.45A'
'He 2 1640.41A' 'O 1 1641.31A' 'C 1 1657.91A' 'O 3 1660.81A'
'O 3 1666.15A' 'Al 2 1670.79A' 'N 3 1749.67A' 'Mg 6 1806.00A'
'Si 3 1882.71A' 'Si 3 1892.03A' 'C 3 1906.68A' 'C 3 1908.73A'
'C 1 1992.01A' 'Si 7 2146.64A' 'O 3 2320.95A' 'C 2 2325.40A'
'C 2 2326.93A' 'Fe 2 2395.63A' 'Fe 2 2399.24A' 'Fe 2 2406.66A'
'Fe 2 2410.52A' 'C 1 2582.90A' 'Fe 2 2598.37A' 'Fe 2 2607.09A'
'Fe 2 2611.87A' 'Fe 2 2613.82A' 'Fe 2 2625.67A' 'Fe 2 2628.29A'
'Mg 7 2628.89A' 'Fe 2 2631.05A' 'Fe 2 2631.32A' 'Mg 5 2782.76A'
'Mg 2 2795.53A' 'Mg 2 2802.71A' 'Fe 4 2829.36A' 'Fe 4 2835.74A'
'Ar 4 2853.66A' 'Fe 4 3094.96A' 'He 1 3187.74A' 'Ne 5 3345.82A'
'Ne 5 3425.88A' 'Fe 7 3586.32A' 'Fe 6 3662.50A' 'O 2 3726.03A'
'O 2 3728.81A' 'H 1 3734.37A' 'H 1 3750.15A' 'Fe 7 3758.92A'
'H 1 3770.63A' 'H 1 3797.90A' 'H 1 3835.38A' 'Ne 3 3868.76A'
'He 1 3888.64A' 'H 1 3889.05A' 'Fe 5 3891.28A' 'Ne 3 3967.47A'
'H 1 3970.07A' 'S 2 4068.60A' 'S 2 4076.35A' 'H 1 4101.73A'
'Fe 2 4243.97A' 'Fe 2 4276.84A' 'Fe 2 4287.39A' 'Fe 2 4319.62A'
'H 1 4340.46A' 'Fe 2 4346.86A' 'Fe 2 4352.79A' 'Fe 2 4358.37A'
'Fe 2 4359.33A' 'O 3 4363.21A' 'Fe 2 4413.78A' 'Fe 2 4416.27A'
'Fe 2 4452.10A' 'Fe 2 4474.90A' 'Fe 3 4658.05A' 'He 2 4685.68A'
'Fe 2 4814.54A' 'H 1 4861.32A' 'Fe 2 4874.50A' 'Fe 2 4889.62A'
'Fe 2 4905.35A' 'Fe 2 4923.92A' 'Fe 2 4947.39A' 'O 3 4958.91A'
'Fe 2 4973.40A' 'Fe 3 4985.87A' 'Fe 2 5005.52A' 'O 3 5006.84A'
'Fe 2 5018.44A' 'Fe 2 5020.25A' 'Fe 2 5049.30A' 'Fe 2 5072.41A'
'Fe 2 5111.64A' 'Fe 2 5158.01A' 'Fe 2 5158.79A' 'Fe 2 5169.03A'
'Fe 6 5176.04A' 'Fe 2 5184.80A' 'Fe 2 5261.63A' 'Fe 3 5270.40A'
'Fe 2 5273.36A' 'Fe 2 5284.10A' 'Fe 2 5333.66A' 'Fe 2 5376.47A'
'Fe 2 5412.67A' 'Fe 2 5433.15A' 'Fe 2 5527.36A' 'Fe 7 5720.71A'
'He 1 5875.61A' 'He 1 5875.64A' 'Fe 7 6086.97A' 'O 1 6300.30A'
'O 1 6363.78A' 'Fe 2 6516.08A' 'N 2 6548.05A' 'H 1 6562.80A'
'N 2 6583.45A' 'Ni 2 6666.80A' 'He 1 6678.15A' 'S 2 6716.44A'
'S 2 6730.82A' 'Ar 3 7135.79A' 'Fe 2 7155.17A' 'Fe 2 7172.00A'
'Ca 2 7291.47A' 'Ca 2 7323.89A' 'Ni 2 7377.83A' 'Fe 2 7388.17A'
'Ni 2 7411.61A' 'Fe 2 7452.56A' 'Ar 3 7751.11A' 'O 1 8446.25A'
'O 1 8446.36A' 'O 1 8446.76A' 'Cl 2 8578.70A' 'Fe 2 8616.95A'
'Fe 2 8891.93A' 'Fe 2 9051.95A' 'S 3 9068.62A' 'Fe 2 9226.63A'
'H 1 9229.02A' 'Fe 2 9267.56A' 'Fe 2 9399.04A' 'Fe 2 9470.94A'
'S 3 9530.62A' 'H 1 9545.97A' 'H 1 1.00494m' 'S 2 1.02867m'
'S 2 1.03205m' 'S 2 1.03364m' 'He 1 1.08291m' 'He 1 1.08303m'
'H 1 1.09381m' 'O 1 1.12863m' 'O 1 1.12864m' 'O 1 1.12869m'
'O 1 1.12870m' 'O 1 1.12873m' 'Ni 2 1.19102m' 'Fe 2 1.25668m'
'Fe 2 1.27877m' 'H 1 1.28181m' 'Fe 2 1.29427m' 'Fe 2 1.32055m'
'Fe 2 1.32777m' 'Fe 2 1.37181m' 'Fe 2 1.53348m' 'Fe 2 1.59948m'
'Fe 2 1.64355m' 'Fe 2 1.66377m' 'Fe 2 1.67688m' 'Fe 2 1.71113m'
'Fe 2 1.74494m' 'Fe 2 1.79711m' 'Fe 2 1.80002m' 'Fe 2 1.80940m'
'H 1 1.87510m' 'Fe 2 1.89541m' 'Ni 2 1.93877m' 'Fe 2 1.95361m'
'Si 6 1.96247m' 'H 1 2.16553m' 'Si 7 2.48071m']
Accessing specific lines¶
A LineCollection
behaves somewhat like a dictionary, we can access line data by passing strings when we index the LineCollection
. Unlike a dictionary, however, we are not limited to singular keys, we can pass multiple at once in a list
or tuple
to instead return a subset of lines. Not only that, we can also defined composite lines by passing a string of comma separated lines. We demonstrate each of these methods to axis data below.
Note that in reality the data is actually stored in contiguous arrays for efficiency.
Extracting a single line¶
To extract a single line we simply pass the name of the line we want to extract.
[3]:
print(lines["H 1 1215.67A"])
+-------------------------------------------------+
| LINECOLLECTION |
+--------------------+----------------------------+
| Attribute | Value |
+--------------------+----------------------------+
| nlines | 1 |
+--------------------+----------------------------+
| id | 'H 1 1215.67A' |
+--------------------+----------------------------+
| ndim | 1 |
+--------------------+----------------------------+
| nlam | 1 |
+--------------------+----------------------------+
| shape | (1,) |
+--------------------+----------------------------+
| elements | [H, ] |
+--------------------+----------------------------+
| line_ids | ['H 1 1215.67A'] |
+--------------------+----------------------------+
| lam | [1215.67] Å |
+--------------------+----------------------------+
| luminosity | [3.33710923e+34] erg/s |
+--------------------+----------------------------+
| continuum | [2.3214967e+17] erg/(Hz*s) |
+--------------------+----------------------------+
| cont | [2.3214967e+17] erg/(Hz*s) |
+--------------------+----------------------------+
| continuum_llam | [4.70931104e+29] erg/(s*Å) |
+--------------------+----------------------------+
| energy | [10.19883629] eV |
+--------------------+----------------------------+
| equivalent_width | [70861.94139551] Å |
+--------------------+----------------------------+
| lum | [3.33710923e+34] erg/s |
+--------------------+----------------------------+
| nu | [2.46606775e+15] Hz |
+--------------------+----------------------------+
| vacuum_wavelengths | [1215.67] Å |
+--------------------+----------------------------+
Extracting composite lines¶
To extract a composite line (e.g. a doublet or triplet) we pass a string of comma separated lines. This will return a new LineCollection
object with the composite line as the only line in the collection.
[4]:
print(lines["Mg 7 2628.89A, Fe 2 2631.05A"]) # doublet
print(lines["Mg 7 2628.89A, Fe 2 2631.05A, Fe 2 2631.32A"]) # triplet
+-------------------------------------------------------+
| LINECOLLECTION |
+--------------------+----------------------------------+
| Attribute | Value |
+--------------------+----------------------------------+
| nlines | 1 |
+--------------------+----------------------------------+
| id | 'Mg 7 2628.89A, Fe 2 2631.05A' |
+--------------------+----------------------------------+
| ndim | 1 |
+--------------------+----------------------------------+
| nlam | 1 |
+--------------------+----------------------------------+
| shape | (1,) |
+--------------------+----------------------------------+
| elements | [Mg, Fe] |
+--------------------+----------------------------------+
| line_ids | ['Mg 7 2628.89A, Fe 2 2631.05A'] |
+--------------------+----------------------------------+
| lam | [2629.97] Å |
+--------------------+----------------------------------+
| luminosity | [6.7217364e+30] erg/s |
+--------------------+----------------------------------+
| continuum | [4.70482496e+19] erg/(Hz*s) |
+--------------------+----------------------------------+
| cont | [4.70482496e+19] erg/(Hz*s) |
+--------------------+----------------------------------+
| continuum_llam | [2.03921297e+31] erg/(s*Å) |
+--------------------+----------------------------------+
| energy | [4.71428165] eV |
+--------------------+----------------------------------+
| equivalent_width | [0.32962405] Å |
+--------------------+----------------------------------+
| lum | [6.7217364e+30] erg/s |
+--------------------+----------------------------------+
| nu | [1.13990828e+15] Hz |
+--------------------+----------------------------------+
| vacuum_wavelengths | [2630.75425269] Å |
+--------------------+----------------------------------+
+----------------------------------------------------------------------+
| LINECOLLECTION |
+--------------------+-------------------------------------------------+
| Attribute | Value |
+--------------------+-------------------------------------------------+
| nlines | 1 |
+--------------------+-------------------------------------------------+
| id | 'Mg 7 2628.89A, Fe 2 2631.05A, Fe 2 2631.32A' |
+--------------------+-------------------------------------------------+
| ndim | 1 |
+--------------------+-------------------------------------------------+
| nlam | 1 |
+--------------------+-------------------------------------------------+
| shape | (1,) |
+--------------------+-------------------------------------------------+
| elements | [Mg, Fe, Fe] |
+--------------------+-------------------------------------------------+
| line_ids | ['Mg 7 2628.89A, Fe 2 2631.05A, Fe 2 2631.32A'] |
+--------------------+-------------------------------------------------+
| lam | [2630.42] Å |
+--------------------+-------------------------------------------------+
| luminosity | [3.64386398e+31] erg/s |
+--------------------+-------------------------------------------------+
| continuum | [7.06006223e+19] erg/(Hz*s) |
+--------------------+-------------------------------------------------+
| cont | [7.06006223e+19] erg/(Hz*s) |
+--------------------+-------------------------------------------------+
| continuum_llam | [3.05899689e+31] erg/(s*Å) |
+--------------------+-------------------------------------------------+
| energy | [4.71347515] eV |
+--------------------+-------------------------------------------------+
| equivalent_width | [1.19119571] Å |
+--------------------+-------------------------------------------------+
| lum | [3.64386398e+31] erg/s |
+--------------------+-------------------------------------------------+
| nu | [1.13971327e+15] Hz |
+--------------------+-------------------------------------------------+
| vacuum_wavelengths | [2631.20435998] Å |
+--------------------+-------------------------------------------------+
Extracting a subset of lines¶
To extract a subset of lines we pass a list or tuple of the names of the lines we want to extract.
[5]:
print(
lines[("Mg 7 2628.89A", "Fe 2 2631.05A", "Fe 2 2631.32A", "Mg 5 2782.76A")]
)
+-----------------------------------------------------------------------------------------------+
| LINECOLLECTION |
+-------------------------+---------------------------------------------------------------------+
| Attribute | Value |
+-------------------------+---------------------------------------------------------------------+
| nlines | 4 |
+-------------------------+---------------------------------------------------------------------+
| ndim | 1 |
+-------------------------+---------------------------------------------------------------------+
| nlam | 4 |
+-------------------------+---------------------------------------------------------------------+
| shape | (4,) |
+-------------------------+---------------------------------------------------------------------+
| elements | [Mg, Fe, Fe, Mg] |
+-------------------------+---------------------------------------------------------------------+
| line_ids (4,) | [Mg 7 2628.89A, Fe 2 2631.05A, Fe 2 2631.32A, ...] |
+-------------------------+---------------------------------------------------------------------+
| lam (4,) | 2.63e+03 Å -> 2.78e+03 Å (Mean: 2.67e+03 Å) |
+-------------------------+---------------------------------------------------------------------+
| luminosity (4,) | 0.00e+00 erg/s -> 2.97e+31 erg/s (Mean: 9.11e+30 erg/s) |
+-------------------------+---------------------------------------------------------------------+
| continuum (4,) | 2.35e+19 erg/(Hz*s) -> 2.73e+19 erg/(Hz*s) (Mean: 2.45e+19 erg) |
+-------------------------+---------------------------------------------------------------------+
| cont (4,) | 2.35e+19 erg/(Hz*s) -> 2.73e+19 erg/(Hz*s) (Mean: 2.45e+19 erg) |
+-------------------------+---------------------------------------------------------------------+
| continuum_llam (4,) | 1.02e+31 erg/(s*Å) -> 1.06e+31 erg/(s*Å) (Mean: 1.03e+31 erg/(s*Å)) |
+-------------------------+---------------------------------------------------------------------+
| energy (4,) | 4.46e+00 eV -> 4.72e+00 eV (Mean: 4.65e+00 eV) |
+-------------------------+---------------------------------------------------------------------+
| equivalent_width (4,) | 0.00e+00 Å -> 2.91e+00 Å (Mean: 8.93e-01 Å) |
+-------------------------+---------------------------------------------------------------------+
| lum (4,) | 0.00e+00 erg/s -> 2.97e+31 erg/s (Mean: 9.11e+30 erg/s) |
+-------------------------+---------------------------------------------------------------------+
| nu (4,) | 1.08e+15 Hz -> 1.14e+15 Hz (Mean: 1.12e+15 Hz) |
+-------------------------+---------------------------------------------------------------------+
| vacuum_wavelengths (4,) | 2.63e+03 Å -> 2.78e+03 Å (Mean: 2.67e+03 Å) |
+-------------------------+---------------------------------------------------------------------+
Note that this list can include composite lines as well as single lines.
[6]:
print(
lines[("Mg 7 2628.89A, Fe 2 2631.05A", "Fe 2 2631.32A", "Mg 5 2782.76A")]
)
+---------------------------------------------------------------------------------------+
| LINECOLLECTION |
+--------------------+------------------------------------------------------------------+
| Attribute | Value |
+--------------------+------------------------------------------------------------------+
| nlines | 3 |
+--------------------+------------------------------------------------------------------+
| ndim | 1 |
+--------------------+------------------------------------------------------------------+
| nlam | 3 |
+--------------------+------------------------------------------------------------------+
| shape | (3,) |
+--------------------+------------------------------------------------------------------+
| elements | [Mg, Fe, Fe, Mg] |
+--------------------+------------------------------------------------------------------+
| line_ids | ['Mg 7 2628.89A, Fe 2 2631.05A' 'Fe 2 2631.32A' 'Mg 5 2782.76A'] |
+--------------------+------------------------------------------------------------------+
| lam | [2629.97 2631.32 2782.76] Å |
+--------------------+------------------------------------------------------------------+
| luminosity | [6.72173640e+30 2.97169034e+31 0.00000000e+00] erg/s |
+--------------------+------------------------------------------------------------------+
| continuum | [4.70482496e+19 2.35523727e+19 2.72580856e+19] erg/(Hz*s) |
+--------------------+------------------------------------------------------------------+
| cont | [4.70482496e+19 2.35523727e+19 2.72580856e+19] erg/(Hz*s) |
+--------------------+------------------------------------------------------------------+
| continuum_llam | [2.03921297e+31 1.01978363e+31 1.05527233e+31] erg/(s*Å) |
+--------------------+------------------------------------------------------------------+
| energy | [4.71428165 4.71186299 4.45543968] eV |
+--------------------+------------------------------------------------------------------+
| equivalent_width | [0.32962405 2.91404006 0. ] Å |
+--------------------+------------------------------------------------------------------+
| lum | [6.72173640e+30 2.97169034e+31 0.00000000e+00] erg/s |
+--------------------+------------------------------------------------------------------+
| nu | [1.13990828e+15 1.13932345e+15 1.07732057e+15] Hz |
+--------------------+------------------------------------------------------------------+
| vacuum_wavelengths | [2630.75425269 2632.10457459 2783.58110253] Å |
+--------------------+------------------------------------------------------------------+
Line aliases¶
Any of these operations can also be performed by passing some common line aliases defined in the emissions submodule.
[7]:
from synthesizer.emissions import line_aliases
for alias in line_aliases:
print(f"{alias} -> {line_aliases[alias]}")
# We can use any of these aliases in place of the long form ID
print(lines["Hb"])
Hb -> H 1 4861.32A
Ha -> H 1 6562.80A
Hg -> H 1 4340.46A
O1 -> O 1 6300.30A
O2b -> O 2 3726.03A
O2r -> O 2 3728.81A
O2 -> O 2 3726.03A, O 2 3728.81A
O3b -> O 3 4958.91A
O3r -> O 3 5006.84A
O3 -> O 3 4958.91A, O 3 5006.84A
Ne3 -> Ne 3 3868.76A
N2 -> N 2 6583.45A
S2 -> S 2 6730.82A, S 2 6716.44A
+--------------------------------------------------+
| LINECOLLECTION |
+--------------------+-----------------------------+
| Attribute | Value |
+--------------------+-----------------------------+
| nlines | 1 |
+--------------------+-----------------------------+
| id | 'H 1 4861.32A' |
+--------------------+-----------------------------+
| ndim | 1 |
+--------------------+-----------------------------+
| nlam | 1 |
+--------------------+-----------------------------+
| shape | (1,) |
+--------------------+-----------------------------+
| elements | [H, ] |
+--------------------+-----------------------------+
| line_ids | ['H 1 4861.32A'] |
+--------------------+-----------------------------+
| lam | [4861.32] Å |
+--------------------+-----------------------------+
| luminosity | [2.43960665e+33] erg/s |
+--------------------+-----------------------------+
| continuum | [1.31331716e+19] erg/(Hz*s) |
+--------------------+-----------------------------+
| cont | [1.31331716e+19] erg/(Hz*s) |
+--------------------+-----------------------------+
| continuum_llam | [1.66602648e+30] erg/(s*Å) |
+--------------------+-----------------------------+
| energy | [2.55042238] eV |
+--------------------+-----------------------------+
| equivalent_width | [1464.32645177] Å |
+--------------------+-----------------------------+
| lum | [2.43960665e+33] erg/s |
+--------------------+-----------------------------+
| nu | [6.16689414e+14] Hz |
+--------------------+-----------------------------+
| vacuum_wavelengths | [4862.6779924] Å |
+--------------------+-----------------------------+
Getting line ratios¶
In addition to the line extraction methods above, we can also get known ratios by passing the ratio name as an index. These ratios are defined in the line_ratios
submodule (details in the line ratios notebook).
[8]:
ratio = lines["BalmerDecrement"]
print(ratio)
2.9180493435290717
Alternatively, we could use the get_ratio
method. This can also take a ratio name but also enables the passing of a list of two lines to get the ratio of.
[9]:
ratio2 = lines.get_ratio("BalmerDecrement")
ratio3 = lines.get_ratio(["H 1 4861.32A", "H 1 6562.80A"])
print(ratio2)
print(ratio3)
2.9180493435290717
0.34269468479604454
Getting diagnostic diagrams¶
We can also get diagnostic diagrams by passing the name of the diagram as an index. These diagrams are are also defined in the line_ratios
submodule (details in the line ratios notebook).
[10]:
dia = lines["BPT-NII"]
print(dia)
(0.11011948908117017, 3.2777857932958203)
Or we can use the get_diagram
method to get a diagnostic diagram by passing the name of the diagram or a list of lists of lines.
[11]:
dia2 = lines.get_diagram("BPT-NII")
print(dia2)
dia3 = lines.get_diagram(
[["O 3 5006.84A", "H 1 4861.32A"], ["N 2 6583.45A", "H 1 6562.80A"]]
)
print(dia3)
(0.11011948908117017, 3.2777857932958203)
(3.2777857932958203, 0.11011948908117017)
Atrithmetic operations¶
We can perform various arithmetic operations on a LineCollection
object. These operations are performed element-wise on the underlying line data.
[12]:
# Get a subset for the demonstration
lines_subset = lines[("Fe 2 2631.05A", "Fe 2 2631.32A")]
Adding LineCollections¶
We can add two LineCollection
objects together. This will return a new LineCollection
object with the same lines as the two input LineCollection
objects, but with the line data added together.
[13]:
new_line = lines_subset + lines_subset
print(new_line.luminosity / lines_subset.luminosity)
[2. 2.] dimensionless
Scaling a LineCollection¶
We can scale (multiply) a LineCollection
object by a scalar. This will return a new LineCollection
object with the same lines as the input LineCollection
object, but with the line luminosity scaled accordingly.
[14]:
new_lines = lines_subset * 4
print(new_lines.luminosity / lines_subset.luminosity)
[4. 4.] dimensionless
Iterating over a LineCollection¶
We can loop over the individual lines just as we would loop over an array or list. Here we will demonstrate this on a subset.
[15]:
lines_subset = lines[
("Mg 7 2628.89A", "Fe 2 2631.05A", "Fe 2 2631.32A", "Mg 5 2782.76A")
]
for line in lines_subset:
print(
f"ID: {line.id}, Wavelength: {line.lam}, "
f"Luminosity: {line.luminosity}, Continuum: {line.continuum}"
)
ID: Mg 7 2628.89A, Wavelength: [2628.89] Å, Luminosity: [0.] erg/s, Continuum: [2.35015265e+19] erg/(Hz*s)
ID: Fe 2 2631.05A, Wavelength: [2631.05] Å, Luminosity: [6.7217364e+30] erg/s, Continuum: [2.35467231e+19] erg/(Hz*s)
ID: Fe 2 2631.32A, Wavelength: [2631.32] Å, Luminosity: [2.97169034e+31] erg/s, Continuum: [2.35523727e+19] erg/(Hz*s)
ID: Mg 5 2782.76A, Wavelength: [2782.76] Å, Luminosity: [0.] erg/s, Continuum: [2.72580856e+19] erg/(Hz*s)
Computing fluxes¶
By default a line contains the rest frame luminosity and continuum luminosity. If we instead want the flux we can use the get_flux
or get_flux0
methods which each populate the flux
and continuum_flux
attributes on the line. The latter of these will compute the rest frame flux at a distance of 10pc.
[16]:
lines.get_flux0()
print(lines["H 1 4861.32A"].flux, lines["H 1 4861.32A"].continuum_flux)
[2.0389607e-07] erg/(cm**2*s) [1.09763681e+11] nJy
While the former requires an Astropy cosmology object and a redshift to compute the observer frame flux.
[17]:
from astropy.cosmology import Planck18 as cosmo
lines.get_flux(cosmo, 8.0)
print(lines["H 1 4861.32A"].flux, lines["H 1 4861.32A"].continuum_flux)
[3.01857317e-27] erg/(cm**2*s) [1.62499308e-09] nJy
Concatenating LineCollections¶
If rather than adding the values of two LineCollection
objects together we want to instead concatenate them along the first axis, we can use the concatenate
method. This will return a new LineCollection
object with all the lines from the two input LineCollection
objects.
[18]:
import numpy as np
from unyt import Hz, angstrom, erg, s
from synthesizer.emissions import LineCollection
# Simulating having two sets of ndim=2 lines we need to combine
lines1 = LineCollection(
line_ids=["O 3 5006.84A", "H 1 4861.32A"],
lam=[5006.84 * angstrom, 4861.32 * angstrom],
lum=np.array([[1.0, 2.0], [3.0, 4.0]]) * erg / s,
cont=np.array([[0.1, 0.2], [0.3, 0.4]]) * erg / s / Hz,
)
lines2 = LineCollection(
line_ids=["O 3 5006.84A", "H 1 4861.32A"],
lam=[5006.84 * angstrom, 4861.32 * angstrom],
lum=np.array([[5.0, 6.0], [7.0, 8.0]]) * erg / s,
cont=np.array([[0.5, 0.6], [0.7, 0.8]]) * erg / s / Hz,
)
print(lines1.shape, lines2.shape)
lines3 = lines1.concat(lines2)
print(lines3.shape)
(2, 2) (2, 2)
(4, 2)
Extending a LineCollection with new lines¶
If we want to add new lines to a LineCollection
object we can use the extend
method. This will return a new LineCollection
object with all the lines from the original LineCollection
object and the new lines. Note that the shape of the new lines must match the shape of the existing lines.
[19]:
# Set up two line collections with different lines
lines1 = LineCollection(
line_ids=["O 3 5006.84A", "H 1 4861.32A"],
lam=[5006.84 * angstrom, 4861.32 * angstrom],
lum=np.array([[1.0, 2.0], [3.0, 4.0]]) * erg / s,
cont=np.array([[0.1, 0.2], [0.3, 0.4]]) * erg / s / Hz,
)
lines2 = LineCollection(
line_ids=["N 2 6583.45A", "H 1 6562.80A"],
lam=[6583.45 * angstrom, 6562.80 * angstrom],
lum=np.array([[5.0, 6.0], [7.0, 8.0]]) * erg / s,
cont=np.array([[0.5, 0.6], [0.7, 0.8]]) * erg / s / Hz,
)
print(lines1.shape, lines2.shape)
lines3 = lines1.extend(lines2)
print(lines3.shape)
(2, 2) (2, 2)
(2, 4)
Blending lines¶
Lines in a LineCollection
can be blended based on a given wavelength resolution using the get_blended_lines
method. This method takes a set of wavelength bins, and returns a new LineCollection
containing lines blended within each bin.
[20]:
import numpy as np
from unyt import angstrom
# Get a subset of lines to blend
lines_subset = lines["H 1 4861.32A", "O 3 5006.84A", "O 3 4958.91A"]
print("Before blending:")
print(lines_subset)
# Blend the lines onto an arbitrary wavelength grid
lam_bins = np.arange(4000, 7000, 1000) * angstrom
blended_lines = lines_subset.get_blended_lines(lam_bins)
print("After blending:")
print(blended_lines)
Before blending:
+-----------------------------------------------------------------------------------+
| LINECOLLECTION |
+--------------------+--------------------------------------------------------------+
| Attribute | Value |
+--------------------+--------------------------------------------------------------+
| nlines | 3 |
+--------------------+--------------------------------------------------------------+
| ndim | 1 |
+--------------------+--------------------------------------------------------------+
| nlam | 3 |
+--------------------+--------------------------------------------------------------+
| shape | (3,) |
+--------------------+--------------------------------------------------------------+
| available_ratios | [R3, ] |
+--------------------+--------------------------------------------------------------+
| elements | [H, O, O] |
+--------------------+--------------------------------------------------------------+
| line_ids | ['H 1 4861.32A' 'O 3 5006.84A' 'O 3 4958.91A'] |
+--------------------+--------------------------------------------------------------+
| lam | [4861.32 5006.84 4958.91] Å |
+--------------------+--------------------------------------------------------------+
| luminosity | [2.43960665e+33 7.99650802e+33 2.67784170e+33] erg/s |
+--------------------+--------------------------------------------------------------+
| continuum | [1.31331716e+19 1.34528228e+19 1.33876459e+19] erg/(Hz*s) |
+--------------------+--------------------------------------------------------------+
| obslam | [43751.88 45061.56 44630.19] Å |
+--------------------+--------------------------------------------------------------+
| flux | [3.01857317e-27 9.89423625e-27 3.31334607e-27] erg/(cm**2*s) |
+--------------------+--------------------------------------------------------------+
| continuum_flux | [1.62499308e-09 1.66454415e-09 1.65647970e-09] nJy |
+--------------------+--------------------------------------------------------------+
| cont | [1.31331716e+19 1.34528228e+19 1.33876459e+19] erg/(Hz*s) |
+--------------------+--------------------------------------------------------------+
| continuum_llam | [1.66602648e+30 1.60881719e+30 1.63212143e+30] erg/(s*Å) |
+--------------------+--------------------------------------------------------------+
| energy | [2.55042238 2.47629629 2.50023076] eV |
+--------------------+--------------------------------------------------------------+
| equivalent_width | [1464.32645177 4970.42677272 1640.71228946] Å |
+--------------------+--------------------------------------------------------------+
| lum | [2.43960665e+33 7.99650802e+33 2.67784170e+33] erg/s |
+--------------------+--------------------------------------------------------------+
| nu | [6.16689414e+14 5.98765804e+14 6.04553134e+14] Hz |
+--------------------+--------------------------------------------------------------+
| obsnu | [6.85210460e+13 6.65295338e+13 6.71725704e+13] Hz |
+--------------------+--------------------------------------------------------------+
| vacuum_wavelengths | [4862.6779924 5008.23663693 4960.29390118] Å |
+--------------------+--------------------------------------------------------------+
After blending:
+--------------------------------------------------------------------+
| LINECOLLECTION |
+--------------------+-----------------------------------------------+
| Attribute | Value |
+--------------------+-----------------------------------------------+
| nlines | 2 |
+--------------------+-----------------------------------------------+
| ndim | 1 |
+--------------------+-----------------------------------------------+
| nlam | 2 |
+--------------------+-----------------------------------------------+
| shape | (2,) |
+--------------------+-----------------------------------------------+
| available_ratios | [R3, ] |
+--------------------+-----------------------------------------------+
| elements | [H, O, O] |
+--------------------+-----------------------------------------------+
| line_ids | ['H 1 4861.32A, O 3 4958.91A' 'O 3 5006.84A'] |
+--------------------+-----------------------------------------------+
| lam | [4910.115 5006.84 ] Å |
+--------------------+-----------------------------------------------+
| luminosity | [5.11744835e+33 7.99650802e+33] erg/s |
+--------------------+-----------------------------------------------+
| continuum | [2.65208175e+19 1.34528228e+19] erg/(Hz*s) |
+--------------------+-----------------------------------------------+
| cont | [2.65208175e+19 1.34528228e+19] erg/(Hz*s) |
+--------------------+-----------------------------------------------+
| continuum_llam | [3.29779976e+30 1.60881719e+30] erg/(s*Å) |
+--------------------+-----------------------------------------------+
| energy | [2.52507718 2.47629629] eV |
+--------------------+-----------------------------------------------+
| equivalent_width | [1551.77655325 4970.42677272] Å |
+--------------------+-----------------------------------------------+
| lum | [5.11744835e+33 7.99650802e+33] erg/s |
+--------------------+-----------------------------------------------+
| nu | [6.10560971e+14 5.98765804e+14] Hz |
+--------------------+-----------------------------------------------+
| vacuum_wavelengths | [4911.48594295 5008.23663693] Å |
+--------------------+-----------------------------------------------+
Plotting Lines¶
To plot lines you can use a LineCollection
instance’s plot_lines
method. This can be incomprehensible if you plot all lines so we’ll pass a subset to the subset argument.
[21]:
_ = lines.plot_lines(
figsize=(12, 5),
subset=[
"He 2 1025.27A",
"He 2 1084.94A",
"Si 2 1179.59A",
"Si 3 1206.50A",
"He 2 1215.13A",
"H 1 1215.67A",
"Si 2 1264.74A",
"O 1 1302.17A",
"O 1 1304.86A",
],
xlimits=(None, 1350),
ylimits=(10**28.0, 10**36.0),
)
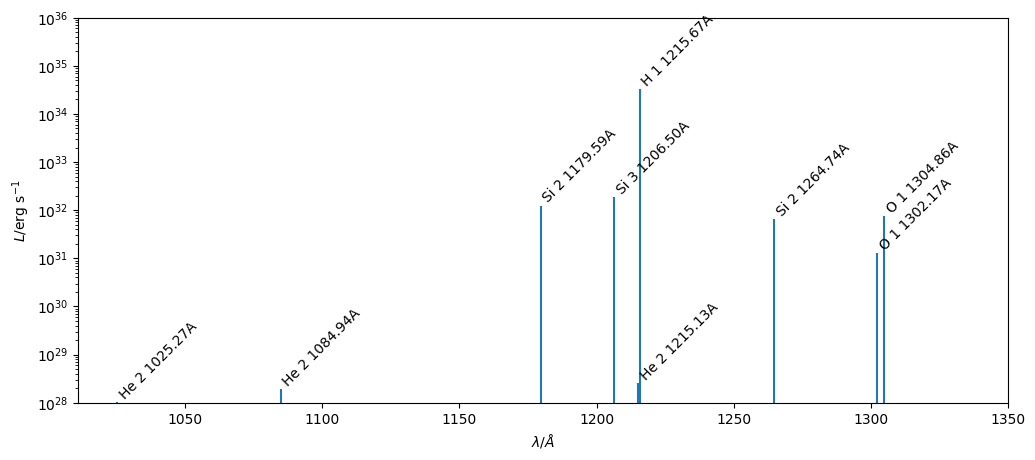